Exceptions in NestJs
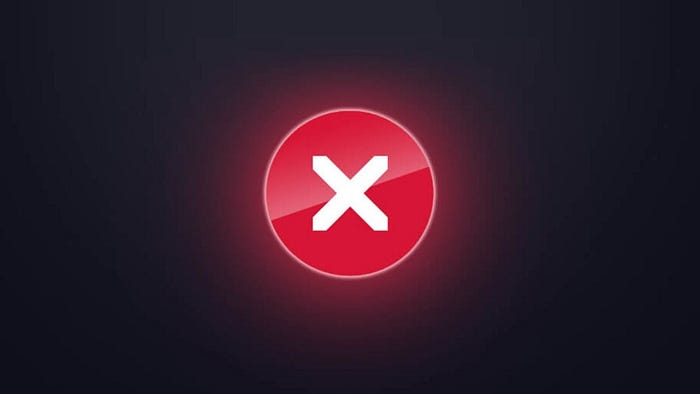
official Implementations: https://docs.nestjs.com/exception-filters
General Exceptions
{
"statusCode": 500,
"message": "Internal server error"
}
Overriding the entire response Body
throw new HttpException({
status: HttpStatus.FORBIDDEN,
error: 'This is a custom message',
}, HttpStatus.FORBIDDEN);//Yields
{
"status": 403,
"error": "This is a custom message"
}
But It still Lacks the Control, so what we can do is create a filter for exception for showing custom response and catching specific response such as Internal server error.
src/common/filter/http-exception.filter.ts
import {
ArgumentsHost,
Catch,
ExceptionFilter,
HttpException,
} from '@nestjs/common';
import { Request, Response } from 'express';export interface ErrorResponse {
timestamp: string;
path: string;
error: {
name: string;
message: Object | string;
};
}@Catch(HttpException)
export class HttpExceptionFilter implements ExceptionFilter {
catch(exception: HttpException, host: ArgumentsHost) {
const ctx = host.switchToHttp();
const response = ctx.getResponse<Response>();
const request = ctx.getRequest<Request>();
let status = exception.getStatus();
let name = exception.getResponse(); let errorResponse: ErrorResponse = {
timestamp: new Date().toISOString(),
path: request.url,
error: {
name: status == 500 ?
'Unknown' :
name['error'] ?
name['error'] :
name,
message: status == 500 ?
'Something Went Wrong' :
exception.message,
},
}; //Finally Send the Modified Response
response.status(
status == 500 ?
400 : status
).json(errorResponse);
}
}
Use This Filter in the Controller Level or the Request Level as required
import {
HttpExceptionFilter
} from 'src/common/filter/http-exception.filter';@UseFilters(new HttpExceptionFilter())
If You want to set it up in the Global Scope then
import { Module } from '@nestjs/common';//Checky Nest JS,Why is this not in the documentation
import { APP_FILTER } from '@nestjs/core';import { AppController } from './app.controller';
import { AppService } from './app.service';
import { HttpExceptionFilter } from './common/filters/http-exception.filter';
import { AppConfigModule } from './config/app/app-config.module';@Module({
imports: [AppConfigModule],
controllers: [AppController],
providers: [
{
provide: APP_FILTER, // <-- See This
useValue: new HttpExceptionFilter(),
},
AppService,
],
})
export class AppModule {}